Text-box¶
The text-box is an output element and displays a multi-line text and manages auto-scrolling.
Configure¶
Warning
Ensure the GUI library is included and initialised according to the configuration procedure.
TextBox myTextBox(&myGUI);
TextBox()
creates a text-box and sets the link to the GUI myGUI
.
1 2 |
|
dDefine()
defines the text-box with vector coordinates.
The required parameters are
- The first line specifies the vector coordinates: top-left coordinates x-y then width and height in pixels.
The optional parameter is
- The second line is optional and specifies the size of the font, by default
0
for the smallest one.
By default, the text-box element is disabled and has no frame.
Then,
myText.setOption(optionWithoutFrame);
myText.setState(stateDisabled);
setOption()
sets a frame for the text-box.
Without and with a frame
Select
-
optionWithoutFrame
orfalse
for text with no frame; -
optionWithFrame
ortrue
for text within a frame.
Note
Use literals instead of values for upward compatibility.
Default is false
for no frame.
setState()
defines whether touch is enabled for the element.
Select
-
stateDisabled
orfalse
for touch disabled; -
stateEnabled
ortrue
for touch enabled.
Note
Use literals instead of values for upward compatibility.
By default, the text-box element is disabled and has no frame.
Use¶
``` cppmyTextBox.draw(“Text”); myTextBox.scroll(); myTextBox.clear();
`draw()` displays the text. If needed, new lines are performed after detected spaces, and auto-scrolling if the last line of the text-box is reached.
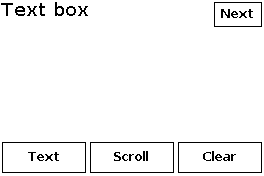
*When the text-box is full, new text automatically scrolls old text up.*
`scroll()` adds a new line. If the last line of the text-box is reached, the whole text is scrolled-up by one line.
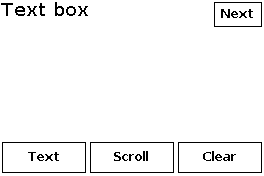
`clear()` clears the text-box.
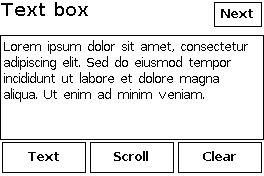
``` cpp
myText.drawFormat("%02i:%02i", 7, 30);
drawFormat()
formats and displays the values, here 07:30
.
Example¶
void displayTextBox()
{
const String string1 = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
const String string2 = "Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam.";
const String string3 = "Quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.";
myScreen.setOrientation(myOrientation);
uint16_t x, y, dx, dy;
x = myScreen.screenSizeX();
y = myScreen.screenSizeY();
dx = x / 3;
dy = y / 5 ;
myGUI.delegate(false);
myGUI.dLabel(0, 0, x, dy, "Text-box", myColours.black, myColours.white, -1, 1, fontLarge);
TextBox myTextBox(&myGUI);
myTextBox.dDefine(0, dy, x, 3 * dy, fontMedium);
myTextBox.setOption(optionWithoutFrame);
myTextBox.draw();
Button myButtonText(&myGUI);
myButtonText.dDefine(0 * dx, 4 * dy, dx, dy, setItem(0x01, "Text"), fontMedium);
myButtonText.setState(stateEnabled);
myButtonText.draw();
Button myButtonScroll(&myGUI);
myButtonScroll.dDefine(1 * dx, 4 * dy, dx, dy, setItem(0x02, "Scroll"), fontMedium);
myButtonScroll.setState(stateEnabled);
myButtonScroll.draw();
Button myButtonClear(&myGUI);
myButtonClear.dDefine(2 * dx, 4 * dy, dx, dy, setItem(0x03, "Clear"), fontMedium);
myButtonClear.setState(stateEnabled);
myButtonClear.draw();
drawNext();
myScreen.flushFast();
myGUI.delegate(true);
uint8_t count = 0;
while (!checkNext())
{
if (myButtonText.check())
{
count++;
count %= 3;
switch (count)
{
case 1:
myTextBox.draw(string1);
break;
case 2:
myTextBox.draw(string2);
break;
default:
myTextBox.draw(string3);
break;
}
}
else if (myButtonScroll.check())
{
myTextBox.scroll();
}
else if (myButtonClear.check())
{
myTextBox.clear();
count = 0;
}
}
}